728x90
반응형
노마드 코더님의 강의 ***‘Flutter 로 웹툰 앱 만들기’***중 pomodoro만드는 것을 보고 작성한 글입니다.
‘뽀모도로'는 이탈리아어로 토마토를 뜻한다. 프란체스코 시릴로가 대학생 시절 토마토 모양으로 생긴 요리용 타이머를 이용해 25분간 집중 후 휴식하는 일처리 방법을 제안한데서 그 이름이 유래했다.
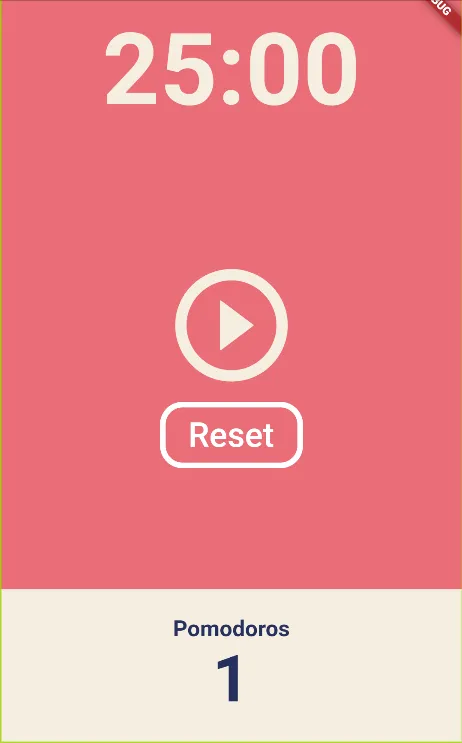
변수 설정
static const twentyFiveMinutes = 1500;
int totalSeconds = twentyFiveMinutes;
bool isRunning = false;
int totalPomodoros = 0;
late Timer timer;
- twentyFiveMinutes : 뽀모도로는 위에 설명한대로 25분을 기준으로 일을 하고 쉬는 것임으로, 25분을 초로 바꾸어서 설정해준다.
- totalSeconds : static 변수로 선언한 값을 totalSeconds에 선언해준다.
- isRunning : 뽀모도로가 시행하는 중인지 중단중인지 알려준다.
- totalPomodoros : 뽀모도로를 시행한 횟수를 말한다.
- timer : Timer를 사용하기 위해 선언한다.
함수 만들기
void onTick(Timer timer) {
if (totalSeconds == 0) {
setState(() {
totalPomodoros = totalPomodoros + 1;
isRunning = false;
totalSeconds = twentyFiveMinutes;
});
timer.cancel();
} else {
setState(() {
totalSeconds = totalSeconds - 1;
});
}
}
void onStartPressed() {
timer = Timer.periodic(const Duration(seconds: 1), onTick);
setState(() {
isRunning = true;
});
}
void onPausePressed() {
timer.cancel();
setState(() {
isRunning = false;
});
}
String format(int seconds) {
var duration = Duration(seconds: seconds);
return duration.toString().substring(2, 7);
}
void onReset() {
if (isRunning == true) {
timer.cancel();
isRunning = false;
}
setState(() {
totalSeconds = twentyFiveMinutes;
});
}
- onTick : timer를 파라미터를 받아온다. 25분이 다 지나면, totalSeconds이 0이 된다.
- setState를 주어 화면에 변화를 준다. totalPomodoros는 횟수 1회 추가해주고, isRunning은 false로 주어 뽀모도로가 중단되었음을 알려준다. 그리고 totalSeconds에 twentyFiveMinutes를 다시 선언해줌으로써 다시 뽀모도로가 돌아갈 수 있게 설정해준다.
- ***timer.cancel()***은 타이머를 중단시켜준다.
- 만약 totalSeconds이 0이 아니라면 뽀모도로가 돌아가는 중이라는 말로, 초를 -1을 해준다.
- onStartPressed
- Timer.periodic 생성자는 아래와 같이 사용할 수 있다. 지정된 간격으로 여러 번 실행해야 하는 코드 블록이 있는 경우에 Timer.periodic 생성자를 사용할 수 있다.
Timer.periodic(Duration duration, void callback(Timer timer))
- isRunning : true로 주어 뽀도모로가 실행되게 한다.
- onPausePressed : 타이머를 중단시틴다. 그리고 isRunning : false로 주어 뽀도모로가 중단되게 한다.
- format : 위에서 25분을 초로 바꾸어 세팅하였다. duration을 print하면 아래와 같다. 그래서 이를 분으로 바꿔줘야 한다. 아래와 같이 subString을 이용하여 분초만 뽑아내어 사용토록 한다.

duration.toString().substring(2, 7)
- onReset : 중간에 뽀모도로를 중단시키면 리셋할 수 있도록 버튼을 생성하였다. 뽀도모로를 실행중에 reset버튼을 누를 경우 timer.cancel()이 되게 하였고, 뽀도모로도 중단되었음을 변수에 선언해주었다. 그리고 화면에 다시 25분이 나타날 수 있도록 setState를 사용하여 25분을 선언해주었다.
🔗 참고
728x90
반응형
728x90
반응형
노마드 코더님의 강의 ***‘Flutter 로 웹툰 앱 만들기’***중 pomodoro만드는 것을 보고 작성한 글입니다.
‘뽀모도로'는 이탈리아어로 토마토를 뜻한다. 프란체스코 시릴로가 대학생 시절 토마토 모양으로 생긴 요리용 타이머를 이용해 25분간 집중 후 휴식하는 일처리 방법을 제안한데서 그 이름이 유래했다.
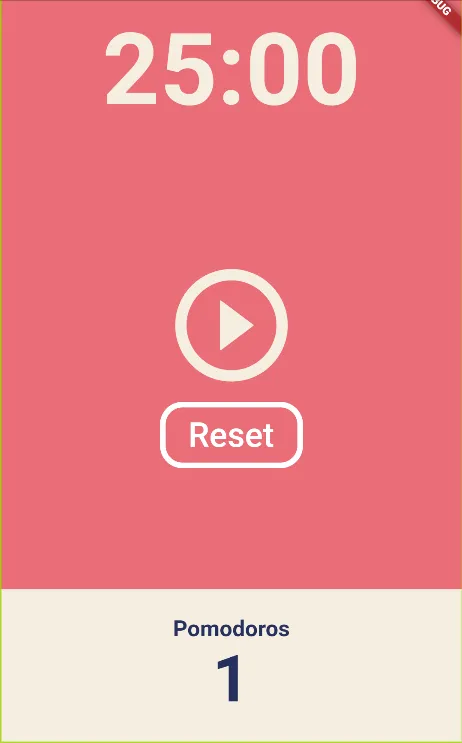
변수 설정
static const twentyFiveMinutes = 1500;
int totalSeconds = twentyFiveMinutes;
bool isRunning = false;
int totalPomodoros = 0;
late Timer timer;
- twentyFiveMinutes : 뽀모도로는 위에 설명한대로 25분을 기준으로 일을 하고 쉬는 것임으로, 25분을 초로 바꾸어서 설정해준다.
- totalSeconds : static 변수로 선언한 값을 totalSeconds에 선언해준다.
- isRunning : 뽀모도로가 시행하는 중인지 중단중인지 알려준다.
- totalPomodoros : 뽀모도로를 시행한 횟수를 말한다.
- timer : Timer를 사용하기 위해 선언한다.
함수 만들기
void onTick(Timer timer) {
if (totalSeconds == 0) {
setState(() {
totalPomodoros = totalPomodoros + 1;
isRunning = false;
totalSeconds = twentyFiveMinutes;
});
timer.cancel();
} else {
setState(() {
totalSeconds = totalSeconds - 1;
});
}
}
void onStartPressed() {
timer = Timer.periodic(const Duration(seconds: 1), onTick);
setState(() {
isRunning = true;
});
}
void onPausePressed() {
timer.cancel();
setState(() {
isRunning = false;
});
}
String format(int seconds) {
var duration = Duration(seconds: seconds);
return duration.toString().substring(2, 7);
}
void onReset() {
if (isRunning == true) {
timer.cancel();
isRunning = false;
}
setState(() {
totalSeconds = twentyFiveMinutes;
});
}
- onTick : timer를 파라미터를 받아온다. 25분이 다 지나면, totalSeconds이 0이 된다.
- setState를 주어 화면에 변화를 준다. totalPomodoros는 횟수 1회 추가해주고, isRunning은 false로 주어 뽀모도로가 중단되었음을 알려준다. 그리고 totalSeconds에 twentyFiveMinutes를 다시 선언해줌으로써 다시 뽀모도로가 돌아갈 수 있게 설정해준다.
- ***timer.cancel()***은 타이머를 중단시켜준다.
- 만약 totalSeconds이 0이 아니라면 뽀모도로가 돌아가는 중이라는 말로, 초를 -1을 해준다.
- onStartPressed
- Timer.periodic 생성자는 아래와 같이 사용할 수 있다. 지정된 간격으로 여러 번 실행해야 하는 코드 블록이 있는 경우에 Timer.periodic 생성자를 사용할 수 있다.
Timer.periodic(Duration duration, void callback(Timer timer))
- isRunning : true로 주어 뽀도모로가 실행되게 한다.
- onPausePressed : 타이머를 중단시틴다. 그리고 isRunning : false로 주어 뽀도모로가 중단되게 한다.
- format : 위에서 25분을 초로 바꾸어 세팅하였다. duration을 print하면 아래와 같다. 그래서 이를 분으로 바꿔줘야 한다. 아래와 같이 subString을 이용하여 분초만 뽑아내어 사용토록 한다.

duration.toString().substring(2, 7)
- onReset : 중간에 뽀모도로를 중단시키면 리셋할 수 있도록 버튼을 생성하였다. 뽀도모로를 실행중에 reset버튼을 누를 경우 timer.cancel()이 되게 하였고, 뽀도모로도 중단되었음을 변수에 선언해주었다. 그리고 화면에 다시 25분이 나타날 수 있도록 setState를 사용하여 25분을 선언해주었다.
🔗 참고
728x90
반응형